@kerry-thacher the action should definitely be there for Flic 2 buttons, as long as they are directly connected to a phone/tablet (not through flic hub).
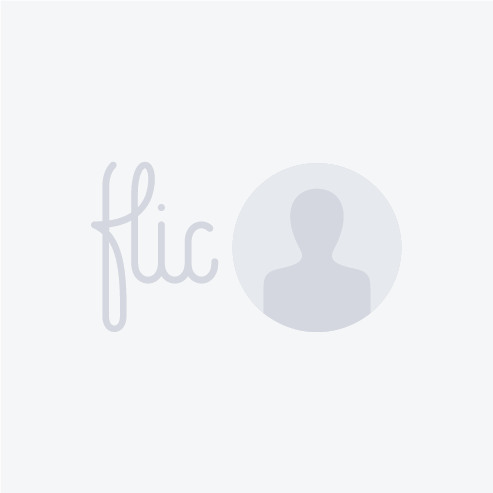
Posts made by Emil
-
RE: Can’t find Flic Universal
-
RE: Flic constantly in standby
@gabrielmgrossman Please file your issue to https://flic.io/support.
-
RE: Play Sonos Groups - Enhancement Request
The problem is that groups can be pretty dynamic. You can hold the play button on one player and it automatically joins another group that is playing music in your household. And with the sonos app it is easy to move around speakers between different groups. That said, it is possible that a group id that was valid when a flic was configured might not be valid after a while after grouping back and forth.
That's why we think it's more simple to pick a player you want to play on, and we will then at runtime when the action is executed check which group that player belongs to and play on that group. May I ask in what circumstances this strategy is a bad idea?
-
RE: Play Sonos Favorite (not Playlist)
@mx5_94 Seems like a very powerful option. We'll definitely implement that!
-
RE: Flic button to emulate keyboard strokes on Android
@SME This works perfectly fine in the Android app. You find the KEYBOARD COMMAND action under the Flic Universal category. There you select "Keys" and then A, B or C.
-
RE: Play Sonos Favorite (not Playlist)
@mx5_94 thanks for the feedback. I assume you think it's more convenient to use sonos favourites over sonos playlists?
-
RE: Flic Hub LR and Home Assistant
@craig-2 we are internally experimenting with our new hub sdk features (https://community.flic.io/topic/18536/new-flic-twist-features-for-the-hub-sdk) together with home assistant (communicating over mqtt) and it works pretty good. So that is one option.
The other option is to expose devices in home assistant as matter devices, as explained here https://community.flic.io/topic/18457/home-assistant-twist-another-angle.
-
RE: New Flic Twist features for the Hub SDK
@mosthated Sorry forgot to mention that only the iOS app shows this provider right now. It will come later to Android.
-
RE: Location permission denied
@bmatest0910 You need to carefully study the readme at https://github.com/50ButtonsEach/flic2lib-android, in particular the section https://github.com/50ButtonsEach/flic2lib-android?tab=readme-ov-file#scanning-for-new-buttons. What API level does your app target and what Android version does your device have?
If targeting and running on Android 12 or higher, ACCESS_FINE_LOCATION is not the permission that you should request at runtime, but BLUETOOTH_SCAN and BLUETOOTH_CONNECT.
If you really need ACCESS_FINE_LOCATION in your app for your other purposes, not Flic-related, such as GPS, you need to follow the instructions at https://github.com/50ButtonsEach/flic2lib-android?tab=readme-ov-file#api-versioning-and-manifest-permissions.
-
RE: Hub vs Hub LR
@beachb_sl the buttons communicate using Bluetooth, not wifi. May I ask you where you have read that they use wifi?
Bluetooth Long Range is a feature introduced in Bluetooth 5.0 (also called Coded PHY) which uses error correcting codes so that the receiver can restore the packet content even if a few bits are lost.
-
RE: Hub vs Hub LR
@beachb_sl yes and of course the main difference that gives the name to Hub LR: Bluetooth Long Range.
-
RE: Hub LR not factory resetting after failed Homekit AND Home Assistant integration attempts
@gerald That is very strange. Could you please shoot a video showing where you hold down the pin hole for 10-15 seconds (not less, not more), also including the LED indicator in the video?
-
RE: Hub LR not factory resetting after failed Homekit AND Home Assistant integration attempts
@gerald Where did you read that you should hold down the pin hole for 15 to 40 seconds? Our manual at https://flic.io/manuals/flichublr states that you need to hold it down for 10 to 15 seconds. Please try this.
-
RE: Crypto for secured requests
@beachb_sl Cryptographic functions are not part of the javascript language itself, but typically included in browser APIs or e.g. Node.js environments.
To use SHA-256 encryption with the Hub SDK, use a pure javascript implementation. You should be able to find that with a simple online search.
If you want to run TypeScript, my suggestion is to transpile it to javascript first.
-
RE: Flic 2 connected in Bluetooth to Alexa
@gianni It appears that alexa skills can only be linked to one account. So you should either use the same flic account for all your flics, or you need to create three amazon accounts and link each to each flic account.
-
RE: Flic 2 connected in Bluetooth to Alexa
@gianni You can assign the Alexa action to as many Flic buttons as you wish. There is no limitation.
-
RE: Listen for device + trigger HTTP request
@claes-jacobsson No idea. I guess you could try to connect to some port maybe. I don't know anything about Apple TV's network usage unfortunately.
-
RE: Listen for device + trigger HTTP request
@claes-jacobsson We have mDNS functionality but it is in beta so it is not documented yet.
But you can refer to the following code samples in the meantime (monitoring Sonos devices):const mdns = require("mdns"); // Service browse: browseHandle = mdns.serviceBrowse(null /*or interface string*/, "_sonos._tcp", function(obj) {console.log(JSON.stringify(obj));}) Output: {"add":true,"interface":"eth0","serviceName":"Sonos-38420B7D0C12@Meeting Room","regtype":"_sonos._tcp.","replyDomain":"local."} {"add":true,"interface":"eth0","serviceName":"Sonos-C43875785444@Kitchen","regtype":"_sonos._tcp.","replyDomain":"local."} {"add":true,"interface":"eth0","serviceName":"Sonos-B8E937B9C4EC@Dev","regtype":"_sonos._tcp.","replyDomain":"local."} {"add":true,"interface":"eth0","serviceName":"Sonos-B8E937EE3C04@Dev","regtype":"_sonos._tcp.","replyDomain":"local."} {"add":true,"interface":"wlan1","serviceName":"Sonos-38420B7D0C12@Meeting Room","regtype":"_sonos._tcp.","replyDomain":"local."} {"add":true,"interface":"wlan1","serviceName":"Sonos-C43875785444@Kitchen","regtype":"_sonos._tcp.","replyDomain":"local."} {"add":true,"interface":"wlan1","serviceName":"Sonos-B8E937B9C4EC@Dev","regtype":"_sonos._tcp.","replyDomain":"local."} {"add":true,"interface":"wlan1","serviceName":"Sonos-B8E937EE3C04@Dev","regtype":"_sonos._tcp.","replyDomain":"local."} browseHandle.cancel(); // Stops the monitoring // Resolve a service instance resolveHandle = mdns.serviceResolve("eth0", "Sonos-38420B7D0C12@Meeting Room", "_sonos._tcp", function(obj) {console.log(JSON.stringify(obj));}); Output: {"interface":"eth0","fullname":"Sonos-38420B7D0C12@Meeting\\032Room._sonos._tcp.local.","hosttarget":"Sonos-38420B7D0C12.local.","port":1443,"txtEntries":{"bootseq":[56,54],"hhid":[83,111,110,111,115,95,76,68,99,69,97,107,111,56,74,56,52,121,102,99,114,54,75,49,89,69,109,48,104,57,99,111],"hhsslport":[49,56,52,51],"info":[47,97,112,105,47,118,49,47,112,108,97,121,101,114,115,47,82,73,78,67,79,78,95,51,56,52,50,48,66,55,68,48,67,49,50,48,49,52,48,48,47,105,110,102,111],"infohash":[52,71,105,56,85,107,108,80,100,68,76,73,112,118,65,99,53,111,75,108,112,87,76,53,102,101,97,110,118,74,106,57,74,73,102,54,95,84,81,109,98,102,103],"location":[104,116,116,112,58,47,47,49,57,50,46,49,54,56,46,51,46,57,58,49,52,48,48,47,120,109,108,47,100,101,118,105,99,101,95,100,101,115,99,114,105,112,116,105,111,110,46,120,109,108],"locationid":[108,99,95,102,57,99,98,52,100,99,54,51,52,55,97,52,53,53,102,56,52,56,97,48,51,101,99,55,52,51,100,52,52,48,98],"mdnssequence":[48],"mhhid":[83,111,110,111,115,95,76,68,99,69,97,107,111,56,74,56,52,121,102,99,114,54,75,49,89,69,109,48,104,57,99,111,46,89,77,77,122,112,67,49,49,72,89,51,79,57,95,110,74,98,70,97,49],"minApiVersion":[49,46,49,46,48],"protovers":[49,46,52,48,46,48],"sslport":[49,52,52,51],"uuid":[82,73,78,67,79,78,95,51,56,52,50,48,66,55,68,48,67,49,50,48,49,52,48,48],"variant":[49],"vers":[53],"wss":[47,119,101,98,115,111,99,107,101,116,47,97,112,105]}} resolveHandle.cancel(); // Stops the monitoring // Get IP address getaddrinfoHandle = mdns.getAddrInfo("eth0", "Sonos-38420B7D0C12.local.", /*IPv4*/true, /*IPv6*/false, function(obj) {console.log(JSON.stringify(obj));}); Output: {"add":true,"interface":"eth0","ip":"192.168.3.9"} getaddrinfoHandle.cancel(); // Stops the monitoring
When you see "add": false, that means the device's dns-sd entry has expired, i.e. the device has not renewed its entry before it expired. You can either monitor all devices of a certain type (serviceBrowse) or monitor a specific host (getAddrInfo).
-
RE: Listen for device + trigger HTTP request
@claes-jacobsson Maybe monitoring it via DNS-SD could be an option. If you use e.g. https://apps.apple.com/us/app/discovery-dns-sd-browser/id305441017, do you see your Apple TV there when you scan your local network?